- Published on
How to print a struct in go (golang)
- Authors
- Name
- Martin Karsten
- @mkdevX
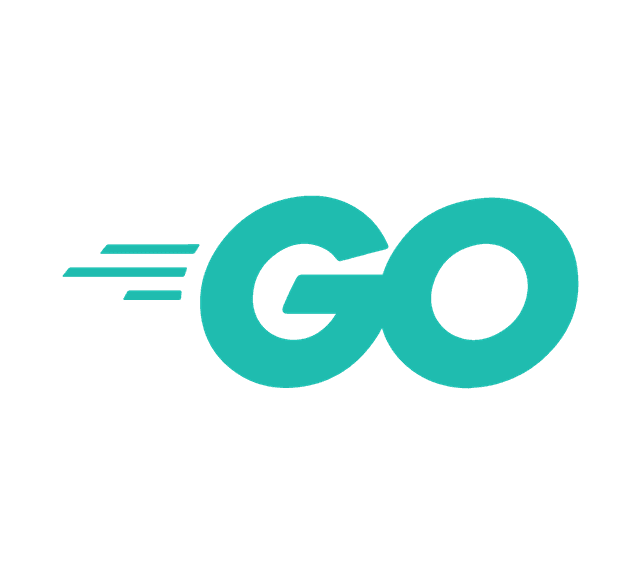
The question of how to print a struct in go (golang) can be quite tricky for new gophers. Often the data will just not appear in your console. For that reason, we will explore how it is done the right way in this post.
Print a struct in your console in go (golang)
Use the fmt.Printf package and add the flag (%+v). This flag adds field names
Code example of printing a struct in go (golang)
import (
"fmt"
)
type MyStruct struct {
Id int64
Title string
Name string
Data Data
}
myStruct := MyStruct{
Id: 1,
Title: "My Title",
Name: "My Name",
}
fmt.Printf("%+v\n", myStruct)
All we have to do is to add %+v to our print function. When printing you will get the following result in your console:
result: {Id:1 Title:My Title Name:My Name}
Print a nested struct in your console in go (golang)
Use the fmt.Printf package and add the flag (%+v). This flag adds field names
Code example on printing a struct in go (golang)
import (
"fmt"
)
type NestedType struct {
OtherId int64
OtherTitle string
OtherName string
}
type MyStruct struct {
Id int64
Title string
Name string
Data NestedType
}
myStruct := MyStruct{
Id: 1,
Title: "My Title",
Name: "My Name",
Data: NestedType{
OtherId: 2,
OtherTitle: "Other Title",
OtherName: "Other Name",
},
}
fmt.Printf("%+v\n", myStruct)
The same logic applies to nested structs. No denesting is necessary. Go's print function in combination with the [%+v]() string will do the denesting in the console for us. When printing you will get the following result in your console:
result: {Id:1 Title:My Title Name:My Name Data:{OtherId:2 OtherTitle:Other Title OtherName:Other Name}}
Print a JSON struct in go (golang)
Use the encoding/json package to json.Marshal the struct and print the string
Code example of printing a JSON object using json.Marshal
import (
"encoding/json"
"fmt"
)
func main() {
type MyStruct struct {
Id int `json:"Id"`
Data []string `json:"Data"`
}
myStruct := MyStruct{
Id: 1,
Data: []string{"Cat", "Dog", "Horse"}}
result, _ := json.Marshal(myStruct)
fmt.Println(string(result))
}
And again, when printing you will get the following result in your console:
{"Id":1,"Data":["Cat","Dog","Horse"]}
Conclusion:
Printing a struct in go (golang) is quite easy. Simply adding the flag (%+v) to your print statement will add the struct fields. For JSON structs we only have to marshal the object and print the generated string.
If you want to know how to pretty print JSON in go (golang), check out this post or this post for the print formatter cheat sheet.